You can find the Matlab Connector on Github. The idea of the connector is to facilitate the use of OKAPI’s API in Matlab. It provides general functions to initialize OKAPI in Matlab, send calculation requests and retrieve the results. It furthermore contains a simple error handling, so you are informed in case something goes wrong.
The whole connector is open source, and you are allowed to do anything you want with it. You can use it to send and retrieve any calculations possible with OKAPI. If you are integrating it into a larger software package, note that OKAPI’s API is currently being set-up and therefore undergoing rapid changes.
This tutorial is split in the following sections:
- Getting the code explains you how to retrieve the code from Github.
- The connector gives a short introduction into the general layout and functionality of the connector. It also gives an overview of the included error handling.
- Step-by-step example helps you to to login and process a request for the very first time.
We suggest to go through the items once, and you should be ready to use the connector.
Getting the code
The code is released on Github. We recommend that you clone the repository from there, as it facilitates staying up-to-date with the API. To clone it, you have to have git installed on your machine. It is available for all operating systems. Generally, git is a command line tool, but for all operating systems also amazing GUIs exist. Furthermore, it is also integrated into Matlab directly.
To clone the repository from the command line, change into a directory of your choice and enter:
git clone https://github.com/OKAPIOrbits/OkapiMatlabConnector.git
The code including the complete repository will now be cloned to that folder and you can start using it. If you are integrating it into a larger software, remember to addpath path_to_connector
to use it. If you want to get the newest version of the repository (for example because you are using a new version of the API), you can get the latest changes by executing:
git pull
from the command line. Note that you have to be in the repository for this to work.
To use the Matlab solution, create a directory, in which you would like to clone the repository. Right click in the current folder window, and choose Source Control --> Manage Files
. A window opens, make sure that you enter the options as shown below (note that you have to choose git as source control integration):
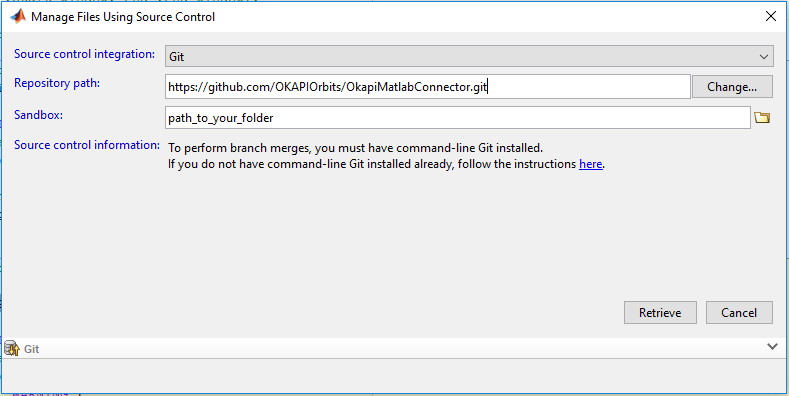
When you click Retrieve, the code is downloaded and included in your folder of choice. If you are integrating it into a larger software, remember to addpath path_to_connector
to use it. To get the newest version of the Matlab connector, again right click in the current folder window and choose Source Control --> Pull
. Git is now download the latest version.
Note: Always make sure that you use the connector in combination with the version of OKAPI you are using. The master of the git-repository is always compatible with the latest release of OKAPI. For all other versions, you find tags of the connector with the same name as OKAPI release. In the future, OKAPI will include a check for the version of both the connector and the version running on the server, but the time, you have to perform this change manually.
The connector
The connector is kept rather simple. All functions are contained in the folder src
of the repository. You will find one routine to perform the log-in (OkapiInit)
, OkapiSendRequest
to start calculations on the server and OkapiGetResult
to retrieve the results from the server, once the calculation has finished.
General use
Generally, the use of the connector is always the same.
- Every 24 hrs (or when re-starting Matlab), you have to get a new JWT token, which is retrieved using
PicardLogin = OkapiInit(<url_to_okapi_as_string>,<your_username_as_string>,<your_password_as_string>);
As url use http://okapi.ddns.net:34568/ or http://okapi.ddns.net:34569/, depending on the version you are calling. The function returns a structPicardLogin
, which contains all information you need to use OKAPI. You do not have to care about it anymore, it simply needs to be passed-on to the other routines. - Then, you have to set-up your request. OKAPI expects the requests as json, in Matlab you can use structs for this. Generally, you have to possibilities: if you have your requests as files, you can read them and convert them to a struct:
Alternatively, you can create the structs in Matlab directly. An example for doing this is given below in the step-by-step example.request_body = jsondecode(fileread(name_of_file_as_string));
- Now everything is set-up, so you can send the result. This is always done using
A list of all available end_point_urls is given in the Api-Documentation. The function call returns two items: a request, and an error struct. More details on the contents of the error struct are given below. The request only contains an id to retrieve your calculation results from the server. You won’t have to do anything with it, but pass it to the function to get the results.[request, error] = OkapiSendRequest(PicardLogin, request_body, end_point_url_as_string);
- Retrieve the results from the server. Note that generally the server needs a moment to process your request. You can tell Matlab to wait using
sleep(seconds_to_sleep)
. Then, you can get the results using
Again, the documentation of all available endpoints to retrieve the results are given in the Api-Documentation. Note that most calculations return the results in different formats. Just choose the format you are interested in. You can also always get the results in all formats available. The function then returns the results of your calculation in the result-struct.[result, error, end_point_url_as_string] = OkapiGetResult(PicardLogin, requests);
Error handling
Until now we have ignored that all functions return an error
object. As you probably do not want your program to crash every time something went wrong, the connector tries to catch the most probable errors that might occur. These errors are then returned to the calling program to decide how to handle them. Overall, the error-struct looks as follows:
error.status = STRING;
error.message = STRING;
error.web_status = integer;
The error.status
contains the overall status of the process. It can contain the values 'NONE'
, 'INFO'
, 'WARNING'
, and 'FATAL'
. The first means that no information on the error state was returned by the server, so everything went fine. The INFO states shows that the server returned some kind of information, which has no impact on the requested calculation. This might be a note that something will be changed in the API interface in a future release. WARNINGs indicate that something happened that might have an impact on the results. In this case you should consult the message for further information and decide, if the warning is relevant for you or not. Last, FATAL means that the calculation could not be finished or the result is known to be wrong. In that case you should not use the returned result for any further calculations. Most often, FATAL states indicate that something went wrong during the communication with the server.
The error.message
gives you some further information on the state.
The error.web_response
give you information on the status of the web request. This is included, as a FATAL state for example can be due to web responses 404, 422, or 500. To identify the error, check out this value. Note that every time something went wrong with the server, you will have FATAL state. So that one should always be the one you handle.
There is one other very important case: it can happen that you request the results from the server before they have been fully processed. In that case, you will get a warning together with a web response 202 (expected). If this is the case, repeat requesting the results until you receive a 200 as http response.
Step-by-step example
Enough with the theory, we know go through one example of using OKAPI step by step. Again, we will use the pass prediction for this. Note that the complete example is contained in the source repository, in the example folder. It will be updated with every release. Anyways, we recommend to go through this example yourself at least once.
Preparations and log-in
At first, you need to tell Matlab were to find the connector functions. You can also work directly work in the src folder of the connector, but this will make it difficult over time to separate between your own and the connector functions. Add the path to the connector using addpath
, in the case of the example contained in the repository it looks like this:
addpath ../src/
Second step is to perform the log-in. This is just a simple call of the function OkapiInit
. The function returns a PicardLogin
, which you can use from now for all server communications:
Note that to use the correct port for the version you are using (the current port fits to the most recent version of OKAPI).
PicardLogin = OkapiInit('http://okapi.ddns.net:34568/',your_username_as_string,your_password_as_string);
Preparing a request
Now, you have to set-up the request to send it to OKAPI. As explained above, there are different ways. The description of the request-formats is given in the Api-Documentation. Note that we are currently introducing a “generic” API, which allows more flexible data types, which will be the standard in the future. For the start, you can just copy the request given here and save it to a file:
{
"tle":"1 25544U 98067A 18218.76369510 .00001449 00000-0 29472-4 0 9993\n2 25544 51.6423 126.6422 0005481 33.3092 62.9075 15.53806849126382",
"simple_ground_location":{
":10.645,
"latitude":52.3283,
"altitude":0.048
},
"time_window":{
"start":"2018-08-07T18:00:00.000Z",
"end":"2018-08-08T00:00:00.000Z"
}
}
You can then read this file directly to the correct fileformat using Matlab’s jsondecode and fileread functions:
RequestBody = jsondecode(fileread(name_of_file_as_astring));
Note that the TLE string contains a newline character. When reading from file it is translated correctly. But, in case you are setting up the struct yourself in Matlab, you need to use a different character. Here is an example how to set-up the a correct struct for the request. Note the newline
in the TLE:
groundLocation = struct('longitude',10.645,'latitude',52.3283,'altitude',0.048);
timeWindow = struct('start','2018-08-07T18:00:00.000Z','end','2018-08-08T00:00:00.000Z');
tle = ['1 25544U 98067A 18218.76369510 .00001449 00000-0 29472-4 0 9993' newline '2 25544 51.6423 126.6422 0005481 33.3092 62.9075 15.53806849126382'];
RequestBody = struct('tle',tle, 'simple_ground_location', groundLocation, 'time_window', timeWindow);
Sending a request
Once the request is prepared, it can be send to OKAPI. There are different end points available to send pass prediction requests. We use the most basic pass/predictions
:
[result, OkapiError] = OkapiSendRequest(PicardLogin, request, 'pass/predictions');
This will send the request to the server, and return request and error structs. Before looking at the request, you should handle the error:
if (strcmp(OkapiError.status, 'FATAL'))
% do something about fatal errors
error(OkapiError.message);
elseif (strcmp(OkapiError.status, 'WARNING'))
% do something about warnings
display(OkapiError.message);
end
In this example, the script is terminated in case a fatal error occurred. The error together with all other information available is printed to the screen. In case of warnings, the warning is shown but the script continues to run. You can of course decide on other actions for different types of errors.
Getting the result
Now the calculation is running on the server. Give it a short moment to perform the calculations. In Matlab, you can wait using pause(2)
, which should be enough for the pass prediction. To get the results, call:
[result, OkapiError] = OkapiGetResult(PicardLogin, request, 'pass/predictions');
Remember to check for errors. In case the result has not been fully processed yet, you will get a 202 as response. In that case, try sending the request again. The result struct then contains your processed request, in the format as described in the Api-Documentation. Especially if you are running calculations that take longer times to process (for example numerical propagations), consider getting the results in a while loop (with pauses in between), until the http response returns a 200. Do avoid DDOS attacks, you should also include a pause in that loop.